SK open API
Recopick 레코픽은 성능과 안정성이 검증된 실시간 개인화 추천엔진과 SK그룹사 데이터를 기반으로 다양한 개인화 서비스를 제공합니다. SK planet
openapi.sk.com
위 사이트 접속
아래와 같은 화면이 나오면 상단 메뉴 중 [My Project] 클릭
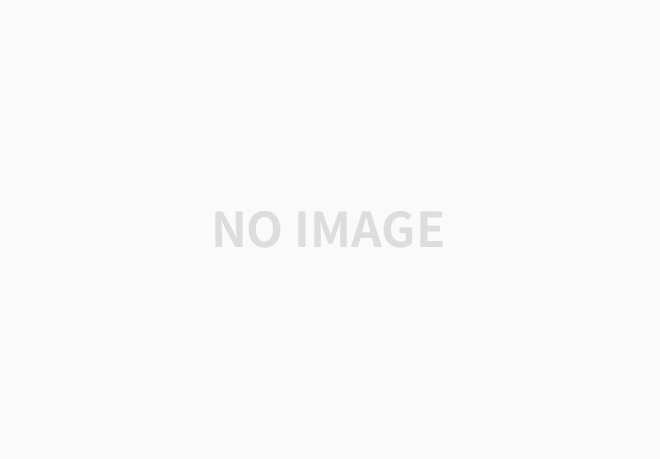
My Project 화면에서 빨간 버튼 [프로젝트 생성] 클릭
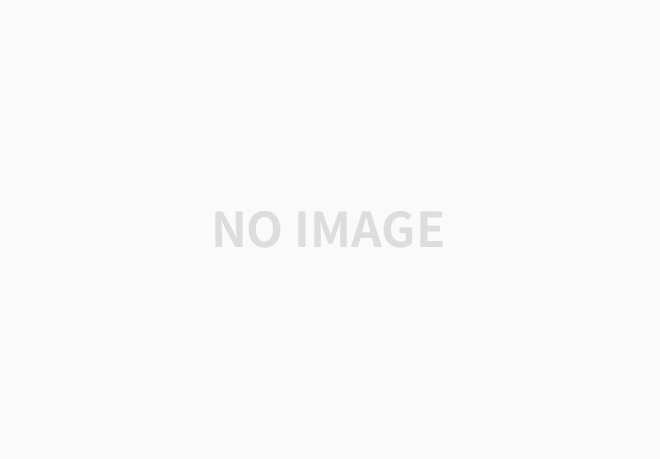
아래와 같은 창이 뜨면 프로젝트명과 설명 입력 후 [확인] 클릭
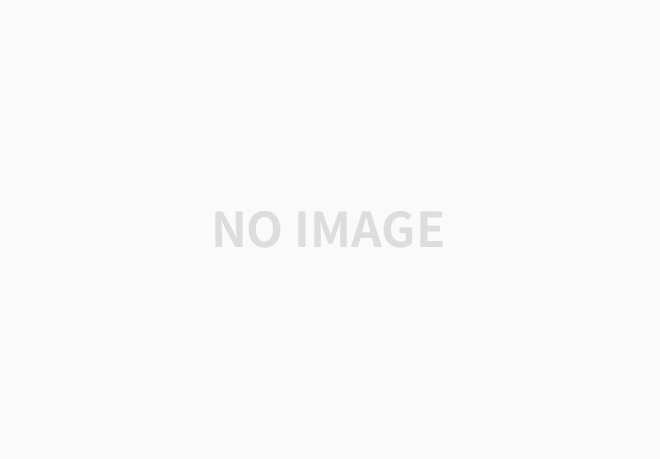
그럼 아래와 같이 내가 생성한 프로젝트가 추가된 것을 확인 가능함.
그럼 이제 상단 메뉴 중 [API] 클릭
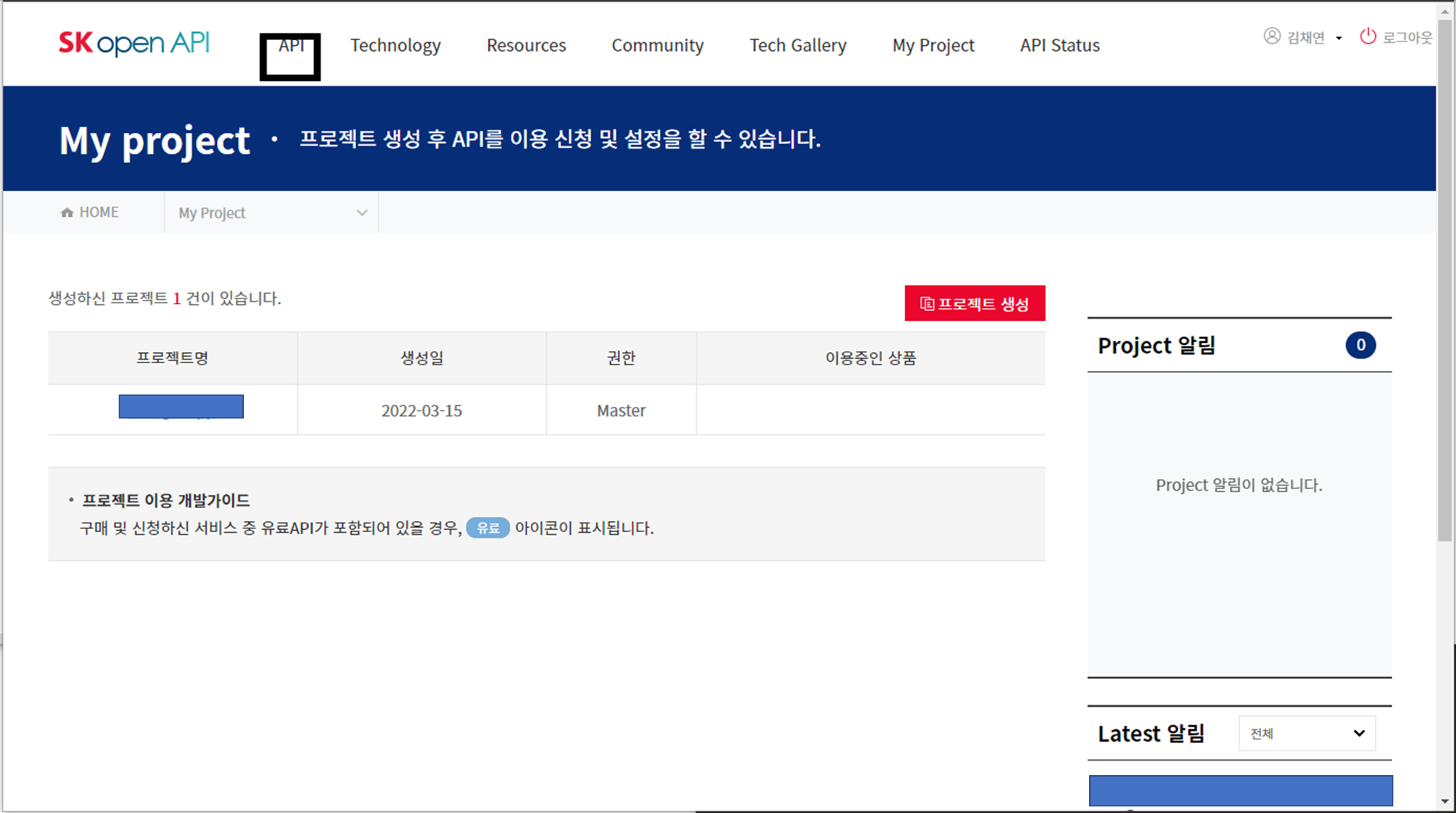
나는 TMAP API를 사용할 것이니 [TMAP] 클릭
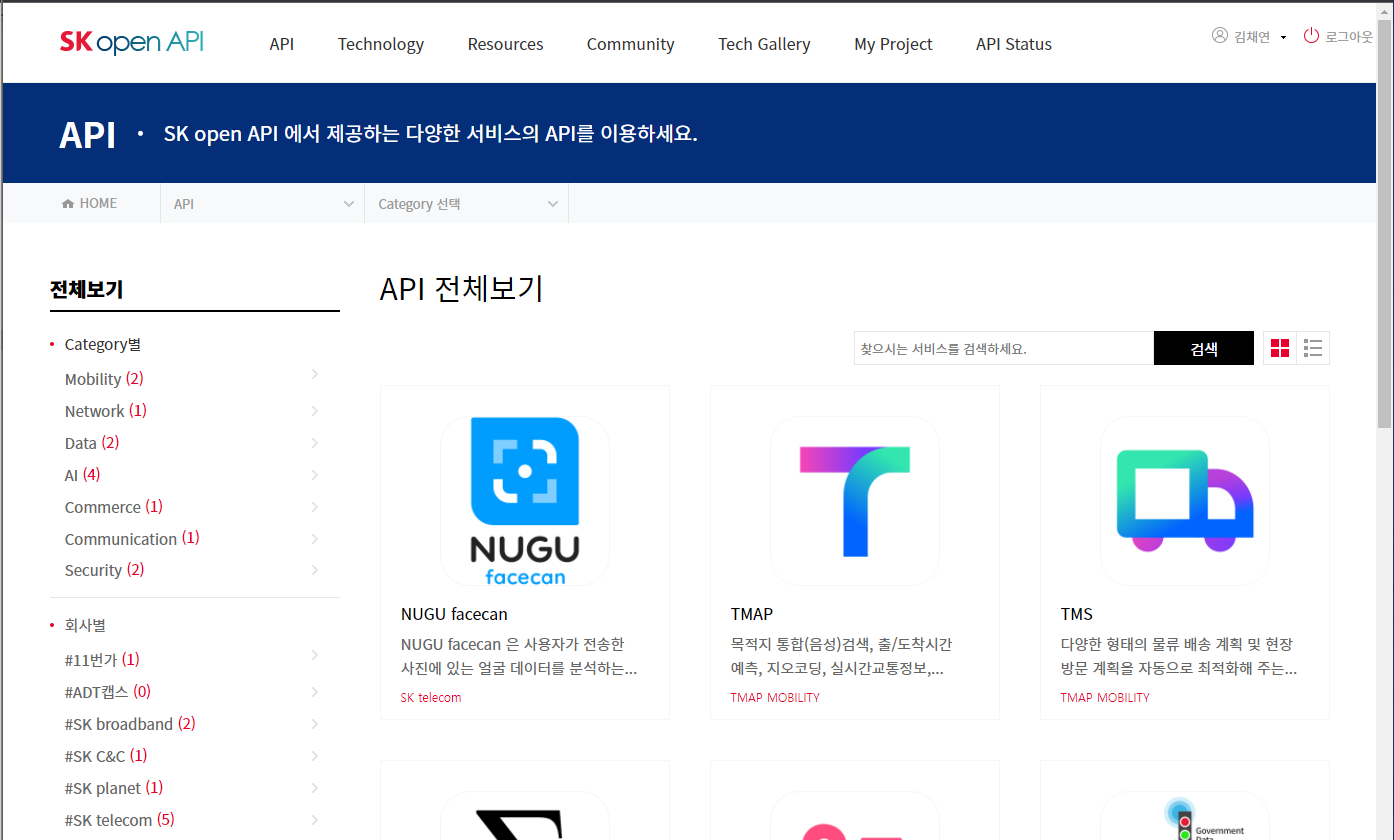
우측에 사용할 API 선택 후 [바로구매] 클릭
TMAP API는 무료.
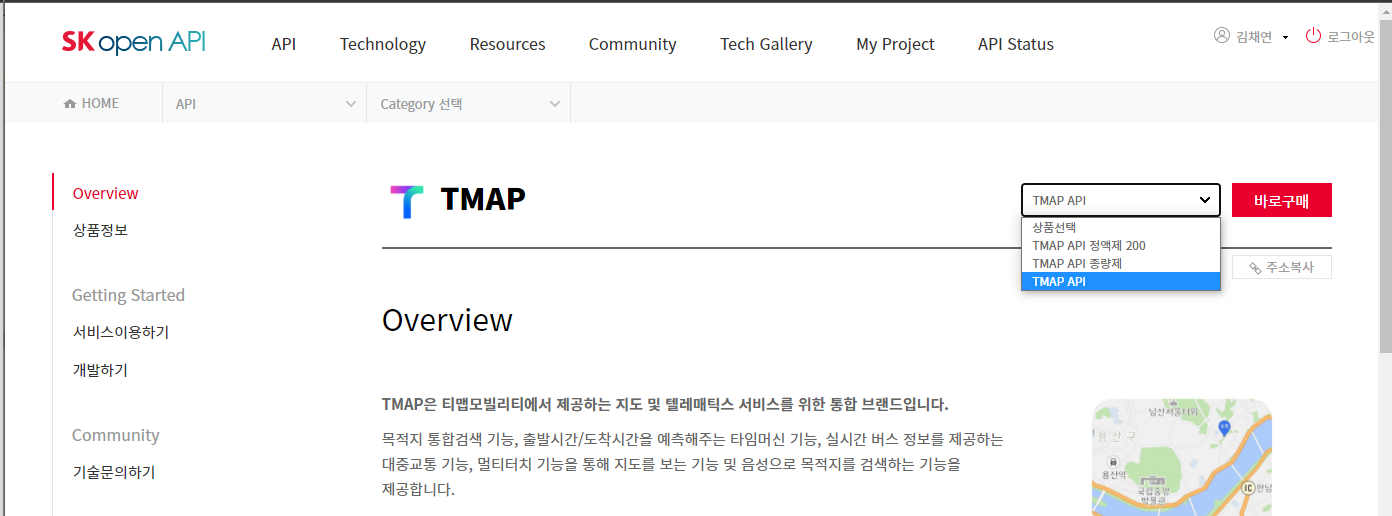
구매를 했다면 다음과 같이 구매 완료 화면이 뜸.
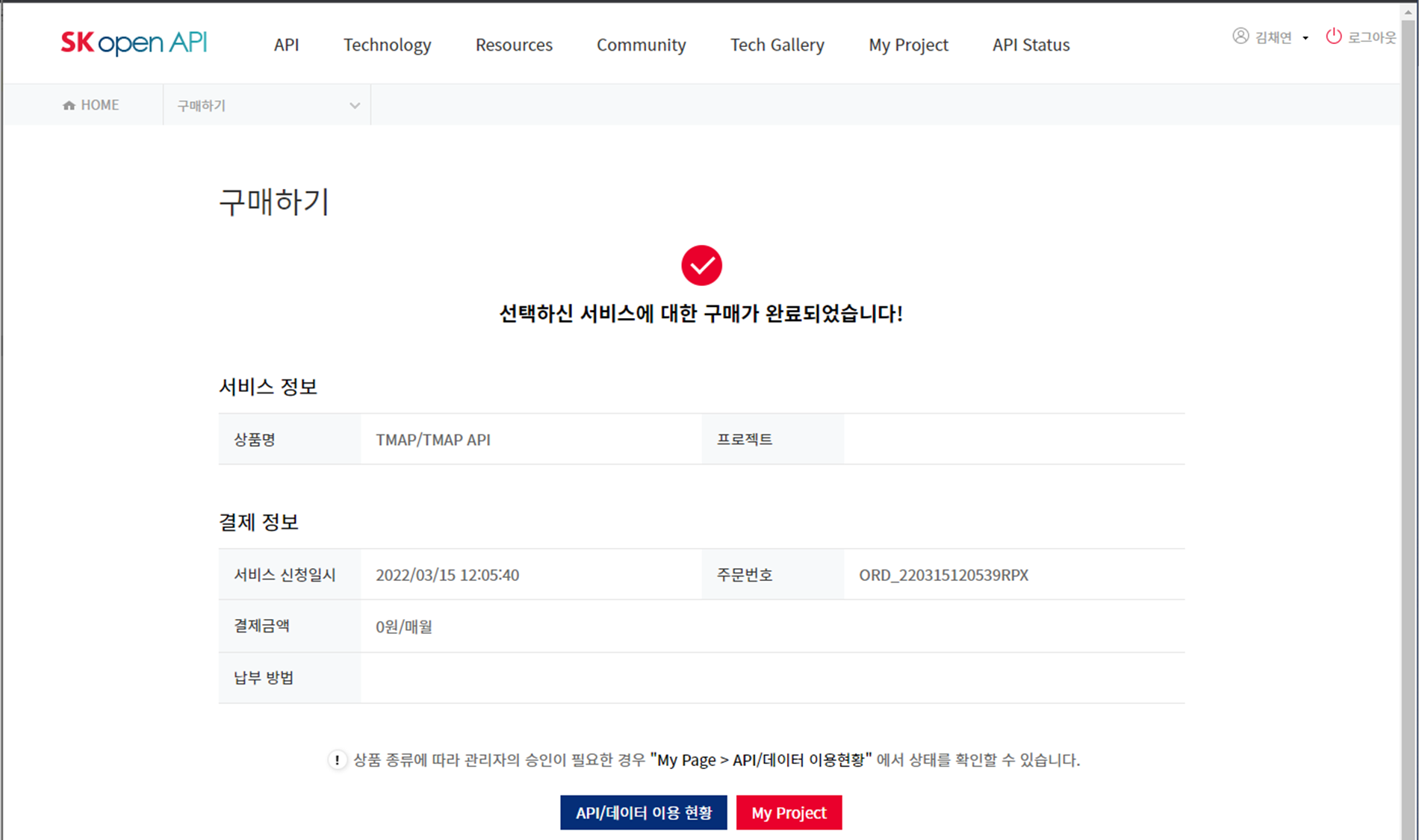
다시 이 화면으로 돌아와서 [자신이 생성한 프로젝트명] 클릭
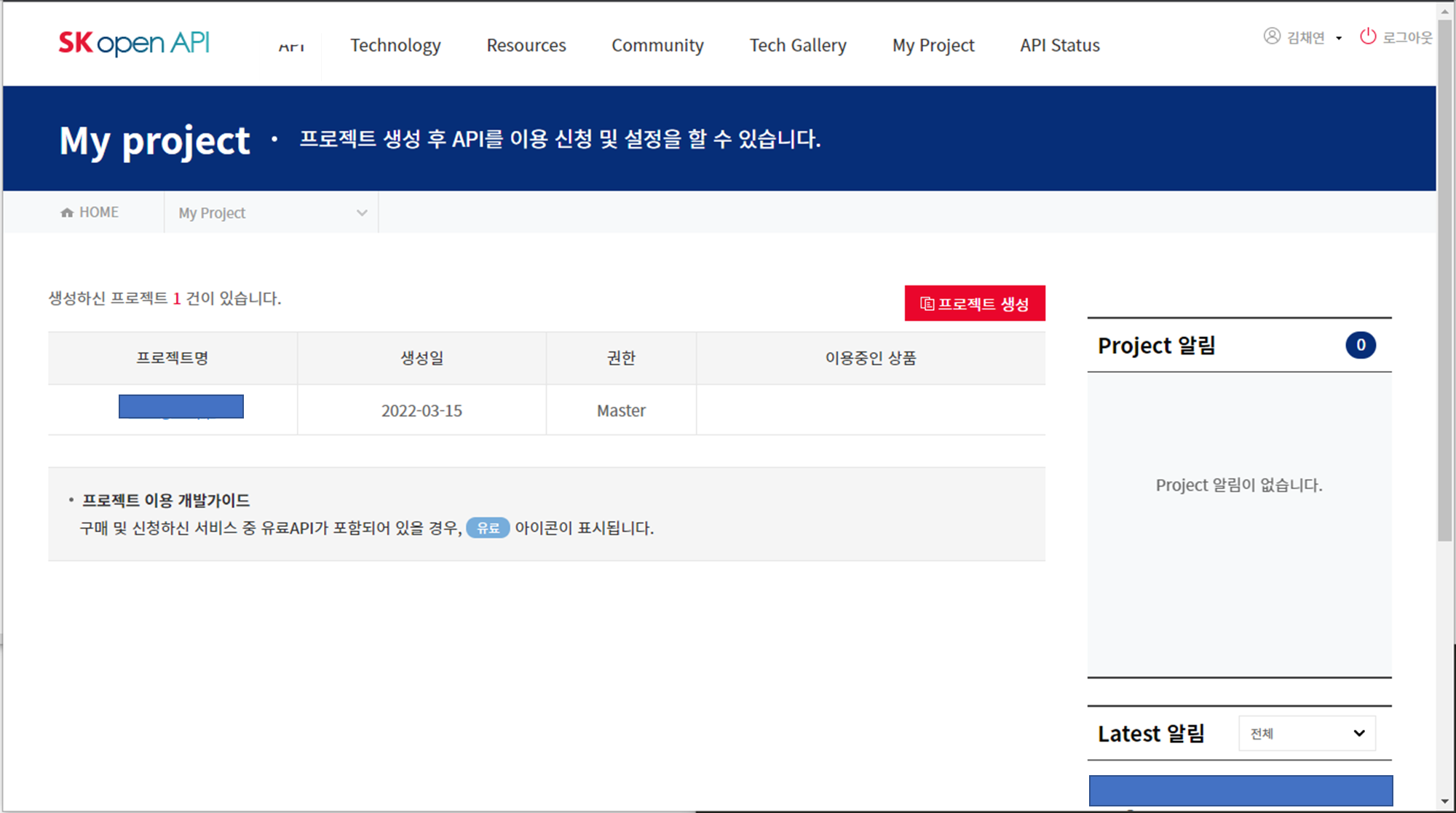
좌측 메뉴들 중 [Key] 클릭
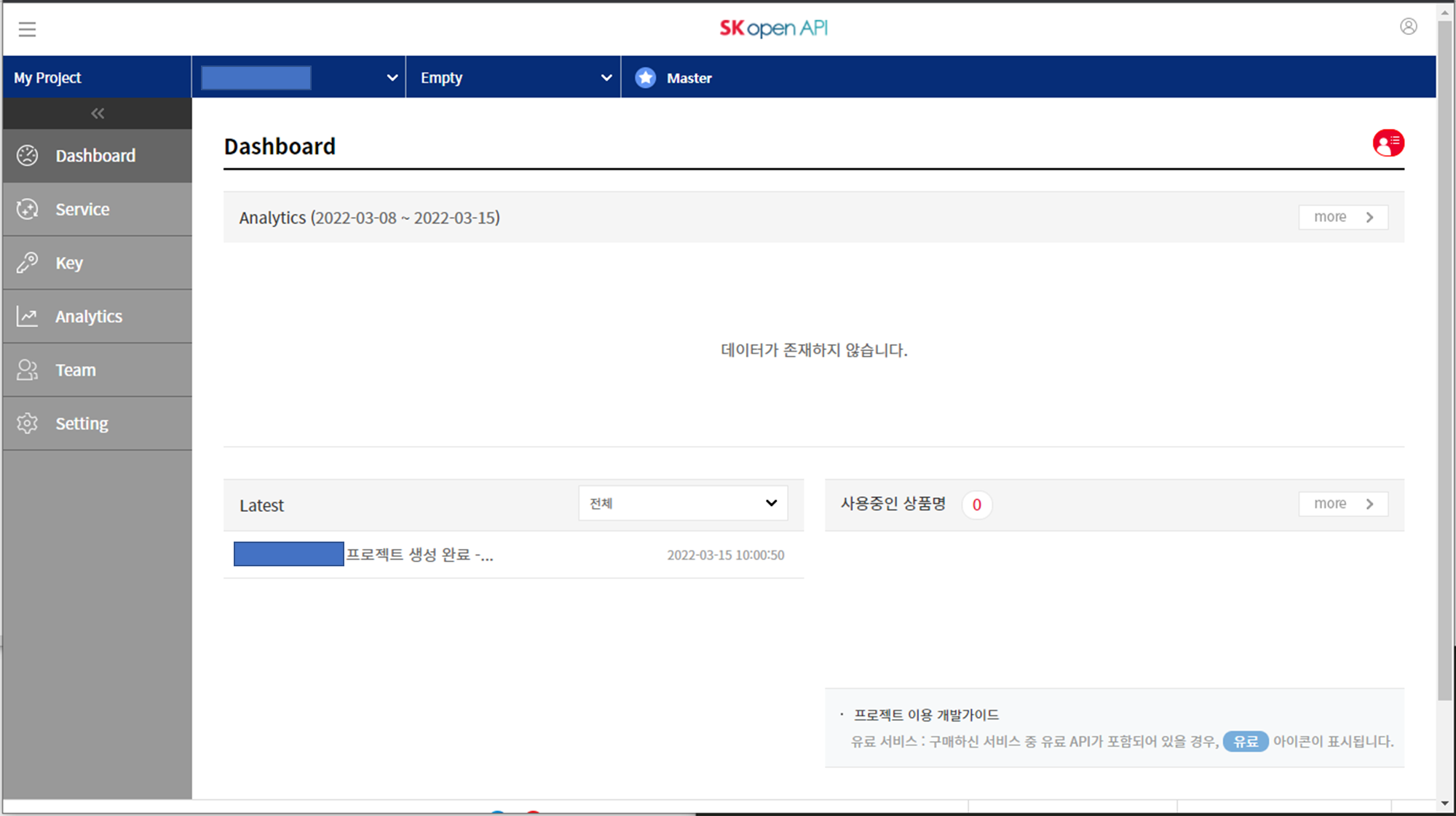
그럼 다음과 같이 나의 App Key를 확인할 수 있음!
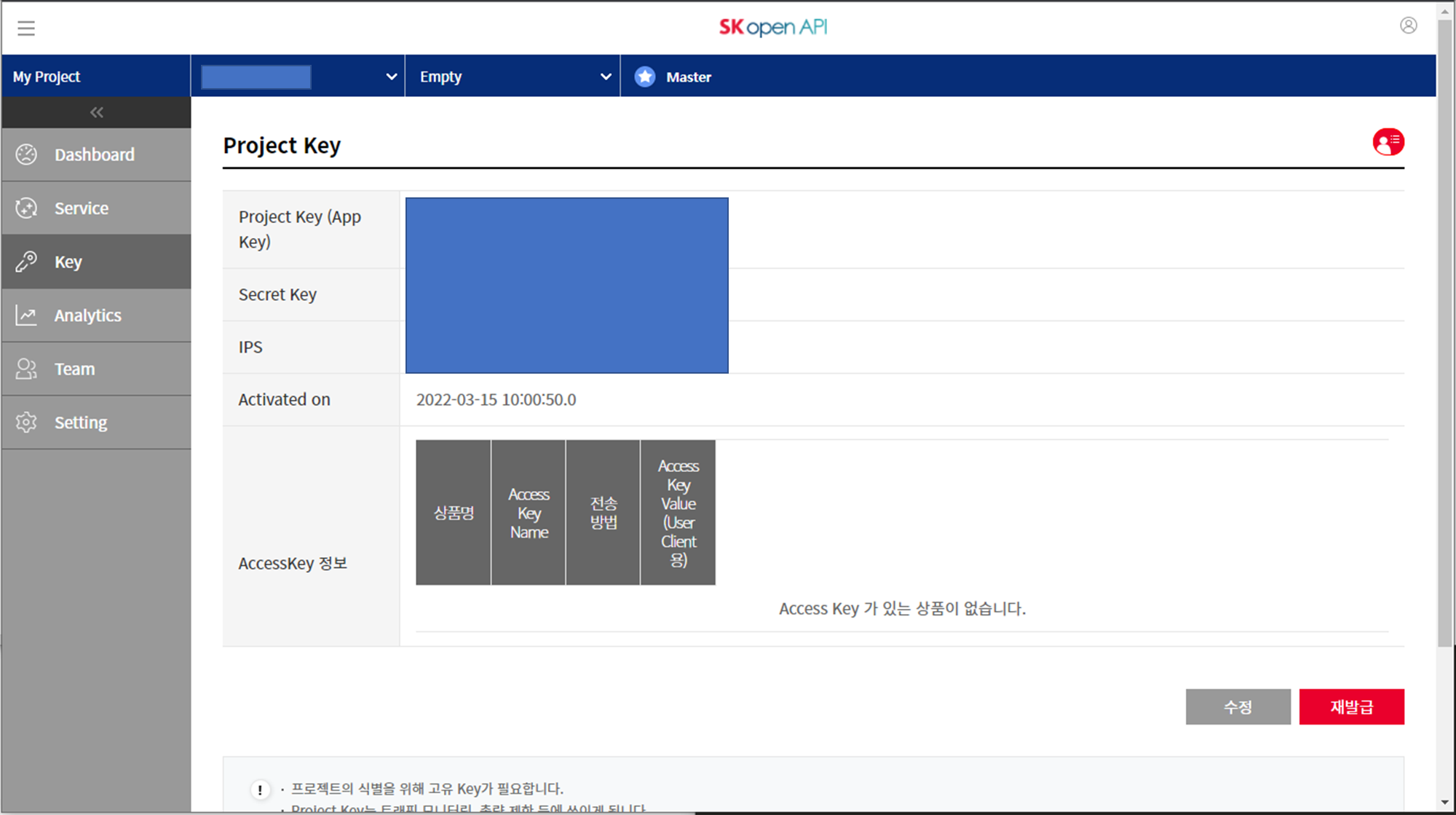
이제 Android SDK를 다운받아 보자.
상단 메뉴들 중 [Resources] 클릭 후 아래 서브 메뉴들 중 [SDK&Tools] 클릭

Android 플랫폼 다운로드 클릭.
나는 iOS도 필요하니 지금 다 다운로드 받음
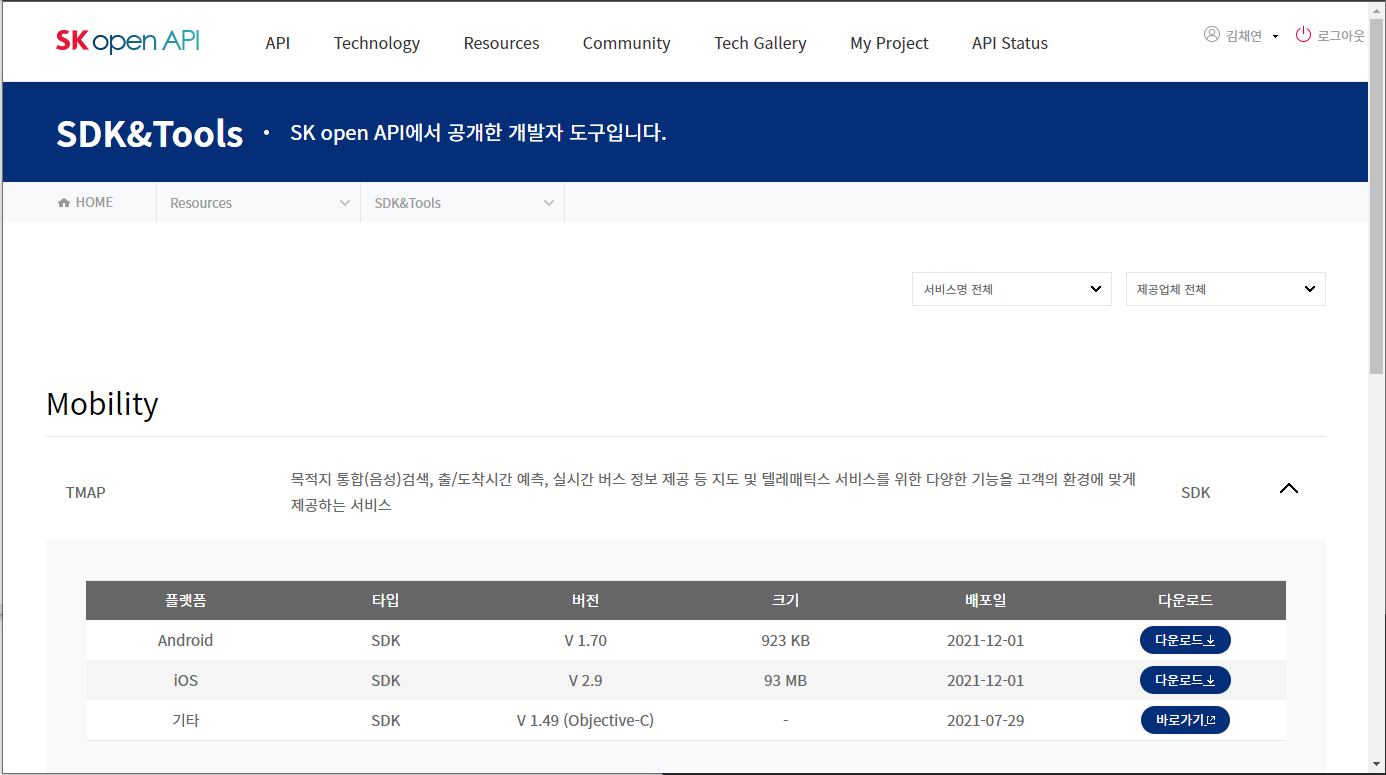
※ 혹시 iOS도 같이 하고 싶다면 아래 포스팅을 참고하면 된다
https://cording-cossk3.tistory.com/212
[Flutter] Tmap API 및 Tmap 앱 연동 (2) - iOS편
후... 반나절을 삽펐다. tmap에서 ios sdk를 다운받고 압축 해제를 하면 아래와 같이 내용물이 들어있다. 혹시 sdk를 어떻게 다운받는지 모른다면 ☞여기😆☜를 클릭해서 보면 된다. 실제 디바이스
cording-cossk3.tistory.com
압축 해제하면 lib 폴더 안에 jar 파일이 존재. 복사
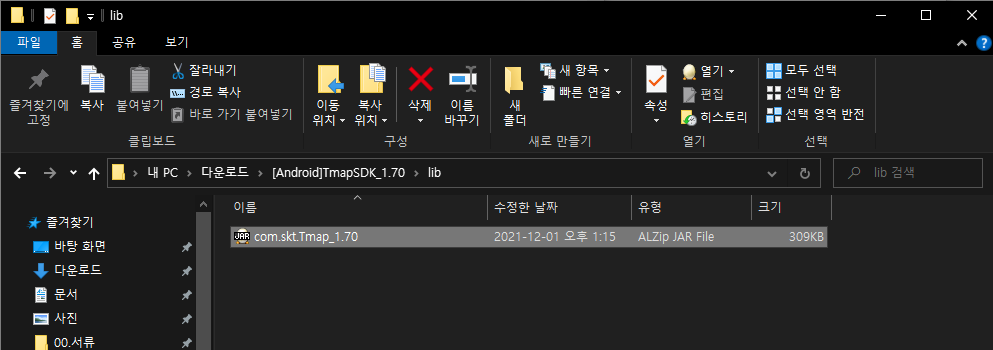
flutter 프로젝트 안 android\app\libs\ 폴더 밑에 붙여넣기
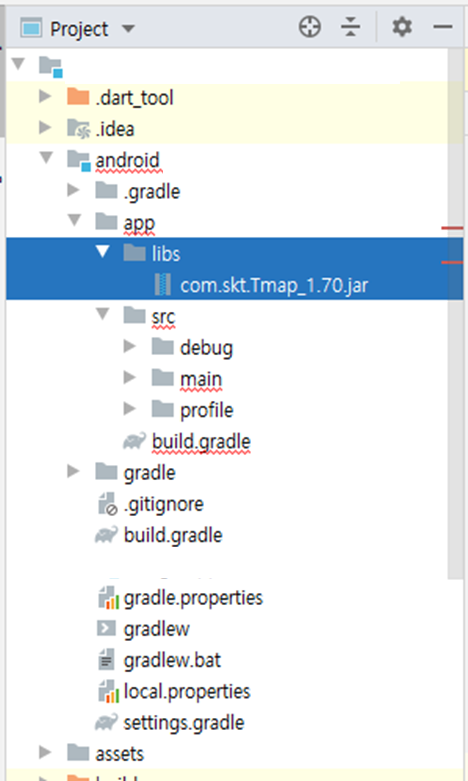
그리고 jar 파일 > 오른쪽 마우스 클릭 > 맨 밑에 [Add As Library...] 클릭
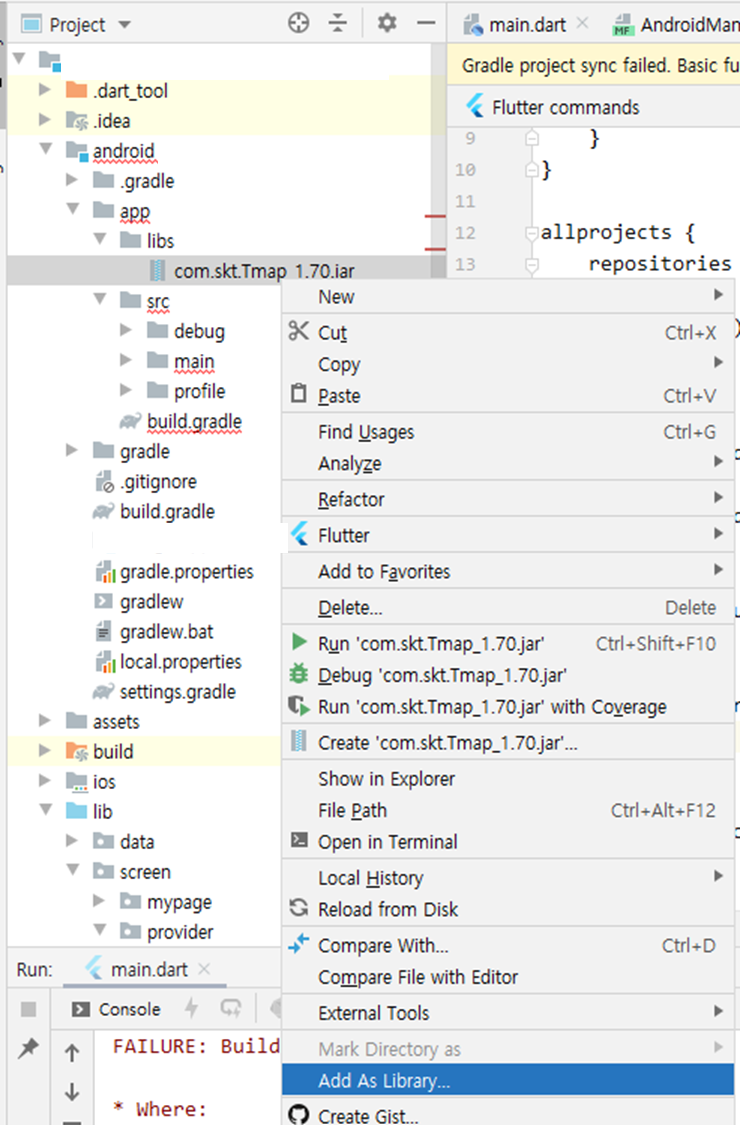
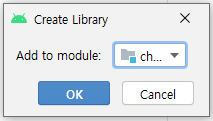
AndroidManifest.xml 에 Internet 권한 추가하기
<manifest>
<uses-permission android:name="android.permission.INTERNET"></uses-permission>
<application>
</application>
</manifest>
MainActivity.java에 flutter에서 호출하기 위한 코드 작성하기
import android.content.Context;
import android.os.Build;
import android.os.Bundle;
import androidx.annotation.NonNull;
import io.flutter.embedding.android.FlutterActivity;
import io.flutter.embedding.engine.FlutterEngine;
import io.flutter.plugins.GeneratedPluginRegistrant;
import io.flutter.plugin.common.MethodChannel;
import io.flutter.plugin.common.MethodChannel.MethodCallHandler;
import io.flutter.plugin.common.MethodChannel.Result;
import io.flutter.plugin.common.MethodCall;
import com.skt.Tmap.TMapTapi;
public class MainActivity extends FlutterActivity {
private static final String mChannel = "mobile/parameters";
@Override
public void configureFlutterEngine(@NonNull FlutterEngine flutterEngine) {
GeneratedPluginRegistrant.registerWith(flutterEngine);
final MethodChannel channel = new MethodChannel(flutterEngine.getDartExecutor(), mChannel);
channel.setMethodCallHandler(handler);
}
private MethodChannel.MethodCallHandler handler = (methodCall, result) -> {
if (methodCall.method.equals("initTmapAPI")) {
TMapTapi tMapTapi = new TMapTapi(this);
tMapTapi.setSKTMapAuthentication("APP KEY 입력");
result.success("initTmapAPI");
} else {
result.notImplemented();
}
};
}
main.dart에서 호출하기
class MyApp extends StatefulWidget {
@override
State<StatefulWidget> createState() => _MyAppState();
}
class _MyAppState extends State<MyApp> {
static const MethodChannel _methodChannel = MethodChannel('mobile/parameters');
Future<void> _initTmapAPI() async {
try {
final String result = await _methodChannel.invokeMethod('initTmapAPI');
print('initTmapAPI result : $result');
} on PlatformException {
print('PlatformException');
}
}
@override
void initState() {
_initTmapAPI();
}
...
}
위의 방법대로 tmap api app key를 인증해준다.
다음은 flutter 앱에서 tmap 앱을 띄워보자
MainActivity.java
private MethodChannel.MethodCallHandler handler = (methodCall, result) -> {
...
else if (methodCall.method.equals("isTmapApplicationInstalled")) {
if (tMapTapi.isTmapApplicationInstalled()) {
result.success("");
} else {
Uri uri = Uri.parse(tMapTapi.getTMapDownUrl().get(0));
Log.e("cylog", "tMapTapi.getTMapDownUrl() : " + tMapTapi.getTMapDownUrl());
result.success(uri.toString());
}
}
...
};
다음과 같이 미설치인 경우, 리턴 값으로 설치 url을 넣어준다.
pubspec.yaml
dependencies:
flutter:
sdk: flutter
# The following adds the Cupertino Icons font to your application.
# Use with the CupertinoIcons class for iOS style icons.
cupertino_icons: ^1.0.2
url_launcher: ^6.0.10
main.dart
Future<void> _tmapCheck() async {
try {
final String result = await methodChannel.invokeMethod('isTmapApplicationInstalled');
print('isTmapApplicationInstalled result : $result');
if (result.isEmpty || result.length == 0) {
//설치
String url =
"https://apis.openapi.sk.com/tmap/app/routes?appKey=APP_KEY_입력&name=SKT타워&lon=126.984098&lat=37.566385";
if (await canLaunch(url)) {
await launch(url, forceSafariVC: false, forceWebView: false);
}
} else {
//미설치 : result = 설치 url
if (await canLaunch(result)) {
await launch(result, forceSafariVC: false, forceWebView: false);
}
}
} on PlatformException {
print('PlatformException');
}
}
같이 보면 좋은 글 ▼
https://cording-cossk3.tistory.com/203
[Flutter] 네이버 Maps API 사용하기
네이버 지도 api 사이트 바로가기 NAVER CLOUD PLATFORM cloud computing services for corporations, IaaS, PaaS, SaaS, with Global region and Security Technology Certification www.ncloud.com 위 링크를 통해 이동하면 아래와 같은 화면
cording-cossk3.tistory.com
https://cording-cossk3.tistory.com/198
[Flutter] Google Map API 사용하기
하나하나 자세하게 알려주는 블로그가 없어 내가 직접 포스팅한다. 우선 Google Cloud Platform으로 이동하자! Google Cloud Platform 이동 Google Cloud Platform 하나의 계정으로 모든 Google 서비스를 Google Cloud Pla
cording-cossk3.tistory.com
https://cording-cossk3.tistory.com/208
[Flutter] Kakao Map api 사용하기
카카오 developer 사이트 바로가기 카카오계정 accounts.kakao.com [ + 애플리케이션 추가하기] 클릭 앱 이름, 사업자명 입력 후 저장 그럼 다음과 같이 리스트에 애플리케이션이 추가된 것을 확인 가능.
cording-cossk3.tistory.com
'프로그래밍 > Flutter-Dart' 카테고리의 다른 글
[Flutter] Tmap API 및 Tmap 앱 연동 (2) - iOS편 (0) | 2022.03.16 |
---|---|
[Flutter] 카카오내비 앱 연동하기 (2) - iOS (0) | 2022.03.16 |
[Flutter] 카카오내비 앱 연동하기 (1) - Android (0) | 2022.03.11 |
[Flutter] Kakao Map api 사용하기 (3) | 2022.03.10 |
[Flutter] Navigator.pop 데이터 전달하기 (0) | 2022.03.10 |
댓글